Following video encoding last time, this time I will take up video decoding. As with encoding, Jetson also includes the NVIDIA Video Decoder Engine (NVDEC), a dedicated hardware accelerator for video decoding. Decoding must be done by this NVDEC for Jetson to work effectively. The APIs available for this are GStreamer and the Jetson Multimedia API, just like for encoding.
[Jetson video processing programming]
Episode 1 What you can do with JetPack and SDK provided by NVIDIA
Episode 2 Video input (CSI-connected Libargus-compliant camera)
Episode 3 Video input (USB-connected V4L2-compliant camera)
Episode 4 Resize and format conversion
Episode 7 Video decoding
Implementation method
Here's how to implement video decoding with NVDEC:
APIs | programming language | comment |
GStreamer |
|
|
Jetson Multimedia API |
|
|
Use GStreamer
Prototyping the GStreamer pipeline
As with encoding, you can prototype the decoding process with the gst-launch-1.0 command.
For example, the pipeline that decodes a file called test.mp4 encoded with the H.264 codec and stored in an MP4 container and displays it on the monitor is as follows.
gst-launch-1.0 -e \ filesrc location=test.mp4 ! \ qtdemux name=demux demux.video_0 ! \ queue ! \ h264parse ! \ nvv4l2decoder ! \ nvegltransform ! \ nveglglessink
GStreamer API
As with encoding, after prototyping on the command line, the GStreamer API integrates into your application.
Using GStreamer from OpenCV
The default OpenCV installed by JetPack does not have a Jetson-specific hardware accelerator enabled, but by using GStreamer as the backend of OpenCV VideoCapture, high-speed video decoding by NVDEC can be used from OpenCV.
You can pass the decoded stream data to OpenCV with the appsink plugin.
import cv2 import sys FILE = 'test.mp4' GST_COMMAND = 'filesrc location={} ! qtdemux name=demux demux.video_0 ! queue ! h264parse ! nvv4l2decoder ! nvvidconv ! video/x-raw,format=(string)I420 ! videoconvert ! appsink'.format(FILE) cap = cv2.VideoCapture(GST_COMMAND) if not cap.isOpened(): print("Cannot open stream") sys.exit() while True: ret, frame = cap.read() if ret == False: break cv2.imshow("Test", frame) key = cv2.waitKey(1) if key == 27: # ESC break cv2.destroyAllWindows() cap.release() sys.exit()
Leverage Multimedia APIs
Video decoding using NVDEC can also be used with the V4L2 Video Decoder included in the Jetson Multimedia API.
Please refer to the sample code included in JetPack for usage. The sample code exists in the following path on Jetson.
/usr/src/jetson_multimedia_api/samples
Next time, we will discuss image processing.
How was the video decoding in Episode 7 of the serial article "Jetson Video Processing Programming"?
In the next section, we will introduce image processing.
If you have any questions, please feel free to contact us.
We offer selection and support for hardware NVIDIA GPU cards and GPU workstations, as well as facial recognition, route analysis, skeleton detection algorithms, and learning environment construction services. If you have any problems, please feel free to contact us.
Related information
![[AI画像解析アプリ開発に必要な知識] 第1話 NVIDIA DeepStream SDKとはのサムネイル画像](/business/semiconductor/articles/134117_thumb_r_18.png)
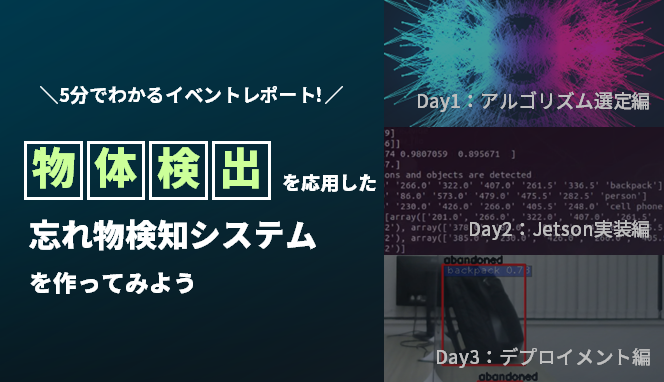
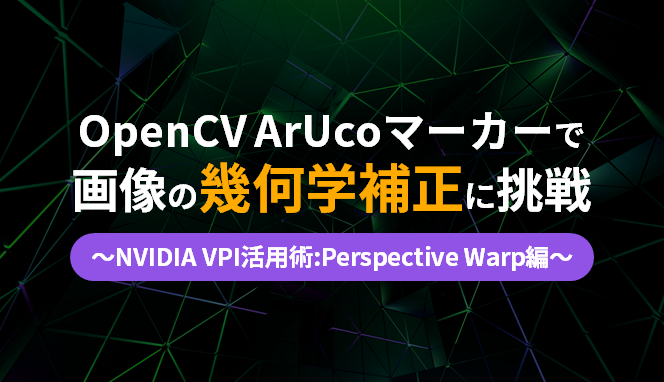
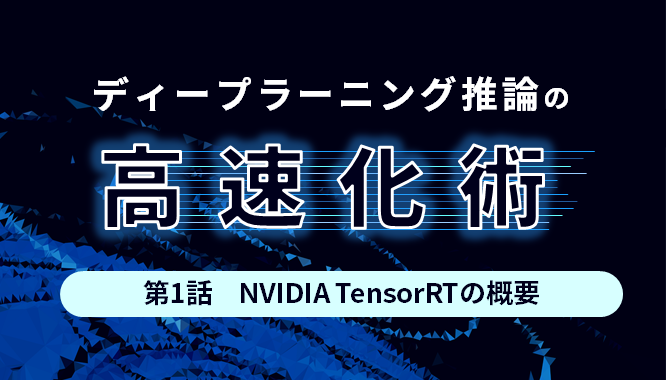