Part 5 of this series will introduce how to display captured images and their processed images on the display.
[Jetson video processing programming]
Episode 1 What you can do with JetPack and SDK provided by NVIDIA
Episode 2 Video input (CSI-connected Libargus-compliant camera)
Episode 3 Video input (USB-connected V4L2-compliant camera)
Episode 4 Resize and format conversion
Episode 5 Image display
Image display API
The following image display APIs can be used with Jetson.
・GStreamer video sink plugin
・OpenCV High-level GUI
・Rendering API provided by Multimedia API
・X11 + OpenGL ES
・NVIDIA Tegra Direct Rendering Manager (DRM)
Each feature is summarized in the table below.
APIs |
programming language |
Strong Points |
Cons/Challenges |
Video sink plugin for GStreamer |
C Python |
|
|
OpenCV High-level GUI |
C Python |
|
|
Rendering API provided by Multitimedia API | C++ |
|
|
X11 + OpenGL ES | C |
|
|
NVIDIA Tegra Direct Rendering Manager (DRM) | C |
|
|
When using GStreamer's video sink plugin
The table below summarizes the video sink plugins that can display image data on NVMM memory (DMA buffers, which can be transferred between hardware modules).
Plugin |
Compatible formats |
remarks |
nv3dsink | RGBA, BGRA, ARGB, ABGR, RGBx, BGRx, xRGB, xBGR, AYUV, Y444, I420, YV12, NV12, NV21, Y42B, Y41B, RGB, BGR, RGB16 | |
nvegltransform + nveglglessink | I420, NV12, I422, NV16, I444, NV24, RGBA |
Need to convert to EGLImage with nvegltranform NVIDIA DeepStream Plugin Manual -> Frequently Asked Questions-> Why is a Gst-nvegltransform plugin required on a Jetson platform upstream from Gst-nveglglessink? |
nvdrmvideosink | Not documented | Ubuntu desktop should be disabled when running |
Plug-ins such as xvimagesink can also be used to display image data on the CPU memory.
When using OpenCV High-level GUI
Image data can be displayed with the imshow function.
Below is a programming example with the OpenCV Python API.
import cv2 import sys cam_id = 0 cap = cv2.VideoCapture(cam_id) if not cap.isOpened(): print("Cannot open camera") sys.exit() while True: ret, frame = cap.read() cv2.imshow("Test", frame) key = cv2.waitKey(1) if key == 27: # ESC break cv2.destroyAllWindows() cap.release() sys.exit()
When using Rendering API provided by Multitimedia API
You can see how to use the Rendering API in the image display part of the V4L2 sample code included in JetPack. This sample code exists in the following path.
/usr/src/jetson_multimedia_api/samples/12_camera_v4l2_cuda
When using X11 + OpenGL ES
The user implements the processing that the Rendering API described above is doing internally. The Rendering API source code is helpful.
/usr/src/jetson_multimedia_api/samples/common/classes/NvEglRenderer.cpp
You can also refer to the following sample code.
/usr/src/jetson_multimedia_api/argus/samples/eglImage
When using NVIDIA Tegra Direct Rendering Manager (DRM)
Please refer to the 08_video_dec_drm sample code provided in the Multimedia API package. Note that this sample code needs to be run after disabling Jetson's Ubuntu desktop. See the instructions on the linked page above for how to do this. The path of the sample code is as follows.
/usr/src/jetson_multimedia_api/samples/08_video_dec_drm
Next time, I will explain how to encode the video!
How did you like the 5th episode of the series "Jetson Video Processing Programming", which introduced how to display the captured image and its processing result image on the display?
Next time, I will introduce how to encode the video.
If you have any questions, please feel free to contact us.
We offer selection and support for hardware NVIDIA GPU cards and GPU workstations, as well as facial recognition, route analysis, skeleton detection algorithms, and learning environment construction services. If you have any problems, please feel free to contact us.
Related information
![[Knowledge required for AI image analysis application development] Episode 1 Thumbnail image of NVIDIA DeepStream SDK](/business/semiconductor/articles/134117_thumb_r_10.png)
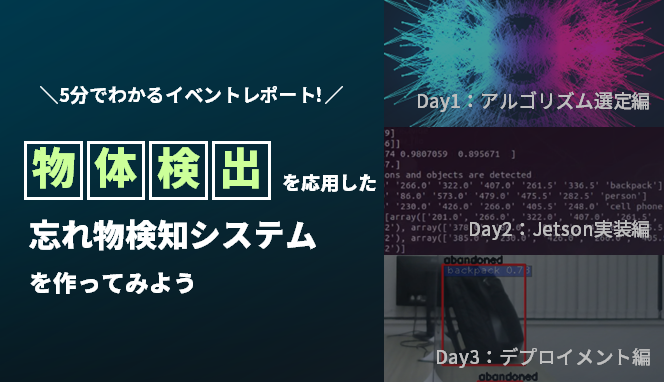
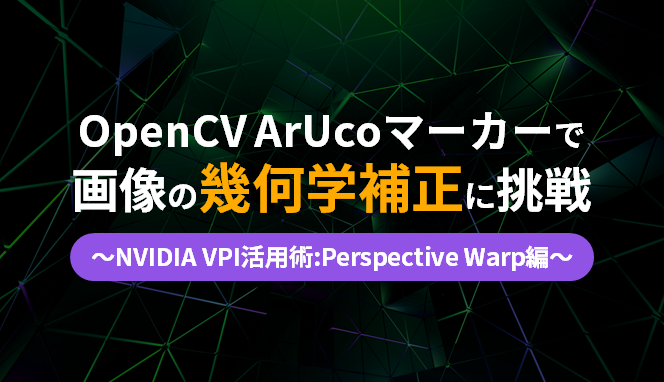
![[Acceleration of deep learning inference] Episode 1 Thumbnail image of the overview of NVIDIA TensorRT](/business/semiconductor/articles/136485_thumb_5.png)